FIRST: make sure you know: how to use What as a String and perform Math expressions . You will not be able to do this assignment until you understand both of the above lessons.
Assign 1) Area of Triangle
Reference packets : #11, #12
Create a world that has a character (person/penguin/whatever) that will ask the user for the height of a triangle and the base of a triangle, and calculate its area
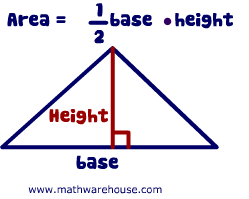
- 3 different variables :
height ,
base and
area of the triangle
- ask the user for a number for the height of the triangle and store it into
height (How to ask user for a number)
- ask the user for a number for the base of the triangle and store it into
base(ask user for a number)
- use area formula and Alice Math expressions to compute the area. (Note: if you don’t know how to use Math, refer to packet 11 or read this post )
- Read this article if you have not already. ( You cannot do step 6 until you have read it)
- Have your character say the area of the triangle (remember
area is a number. You must use what as a string to say it.
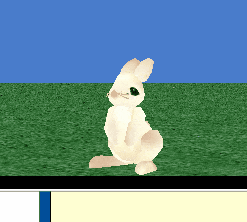
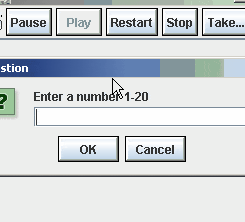
Assign 2) Roman Numeral Translator
Objective : to let the user type in a number between 1 and 10 and then have a character “translate” that into Roman Numerals ( I, II, III, IV, V, VI, VII, VIII, IX, X)
- Ask the user for a number and store the return value into a variable called
numberToTranslate
- use a series of “if/else tests to translate the number and say the appropriate Roman translation for each number between 1 and 10
- Use “==” (link ) to test if two values are the same.
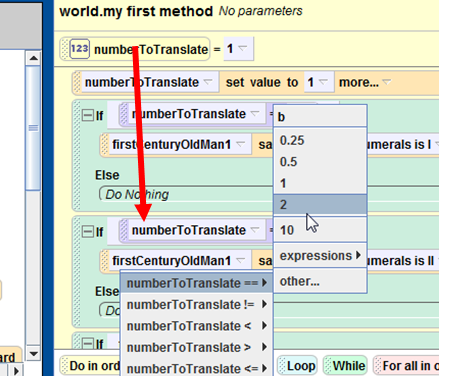
- if the user enters a number greater than 10 then OR less than 1 , have your character say, use either a or b “Only enter numbers between 1 and 10”
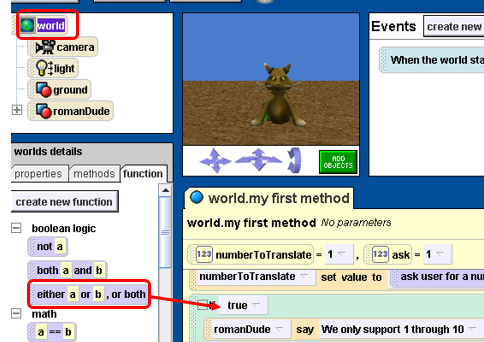
Final result :

We are going to use the roman Numeral Translator file again, so it’s important that you don’t lose the file and give it a good name that you can easily recognize in the future.
Extra credit: Translate 1-20 (Note : You still need to display an error if they enter a number that is too big, which in this case would be >21)
Assign 3) MPG
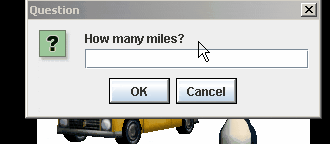
Create a world in which the
- Create 3 variables :
miles, gallons ,MPG
- 2 of the variables store the return value of a function
- The user is asked for the number of gallons of fuel his or her car can hold. Use the return value of the ask user for a number function as shown below.
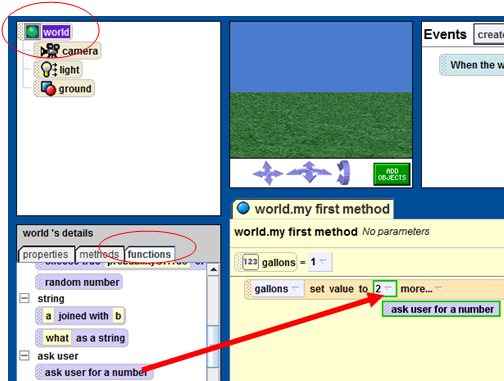
- Do the same thing for the the number of miles that he or she can drive on a full tank. Use ask a user function to set the value of the miles variable.
- a third variable called
MPG . This one will store the result of some math and will hold the car’s miles-per-gallon (MPG). Calculate the MPG with the following formula
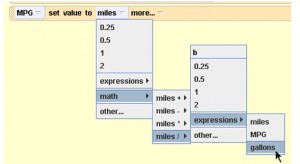
- At the end , your character should
say the value of the
mpg . (You must use what as a string )
Lastly,
- use an if/else as follows
- if mpg is less than 30, say “wow, what a gas guzzler”
- else say “This car gets pretty good gas mileage”
Assign 4) MPG Temp Converter with if/else
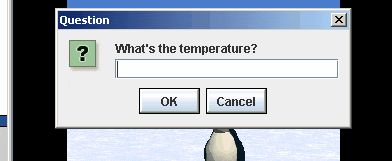
- Create a variable called
degreesFahrenheit .
- Store the return value of the ask user for a number function in
degreesFahrenheit
- Create a variable called
degreesCentigrade
- Use the following formula to convert the
degreesFahrenheit to Centigrade using the following formula below:
|
degreesCentigrade = (5/9) * (degreesFahrenheit − 32) |
- use a character from the
People
collection to say the centigrade temperature.
- Don’t forget that you can’t say numbers, you must use what as a string
- use an if/else so that you say “Wow it’s cold” If the final temperature is
less than 5 and “Whew it’s hot” if the temperature is
greater than 30 (Centigrade)
Assignment 5) Circle’s Area and Circumference: Create a world with your choice of person from the People
collection. When you play the world, it should
- Ask the user for the radius of a circle. (This value should be stored in a variable ).
- Use a circle shape to represent the circle.
- The person should then say the area and the circumference of the circle.( Links for the circumference formula , area of a circle)
- (For an extra point do the same thing with a “Sphere“. Ask the user for the radius and calculate the surface area and the volume.) This should be done in the same file as the circle.
Assignment 5-extra credit ). Look up the formulas for the volume and surface area of a cylinder . Ask the user for the height of the cylinder and the radius, which should be stored in variables. Then calculate the surface area and volume of the cylinder . See if there is a cylinder shape in the gallery to use as a visual aid. And have an object say the volume and surface areas.