In the prior assignment (#2b), you were asked to go around the inner lake as shown below. We are going to add 1 new requirement to this
- with a flower in 6,6
- using while loop(s) traverse around the border of the inner lake
- For each map, you should pick the flower, after the loop is over
What is new this time?
- You are forced to use 1 and only 1 while loop .
- you can only use hop() (ie you can’t hop more than 1 space)
-
after the loop is done , then you can
pick() the flower

Download the map files
recangular-lake.jev
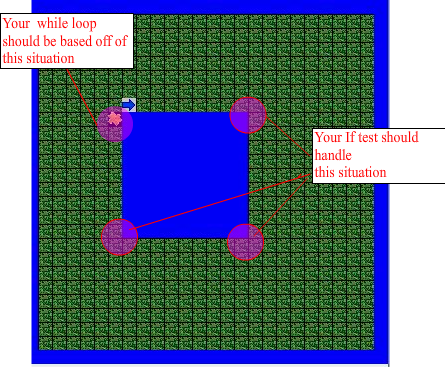
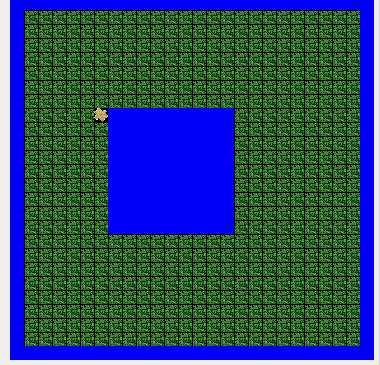
recangular-lake-if2.jev
Scroll down for a hint about how to handle if test #1 for this map.
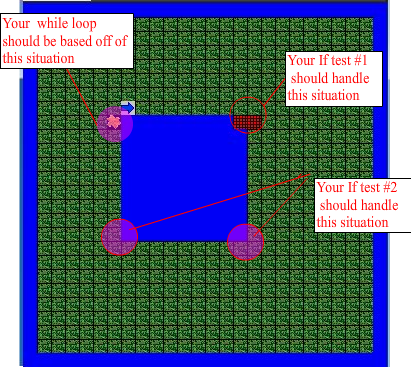
A closerlook at if test #1 (how to get around that first if test)
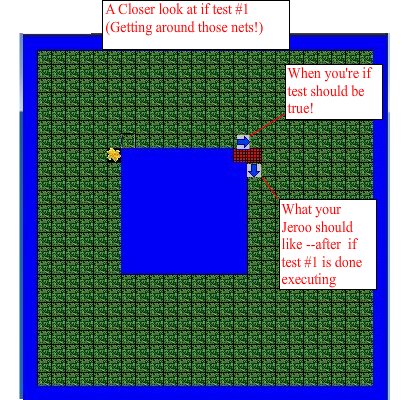
rectangular-lake-bumps1.jev

recangular-lake-bump3A.jev
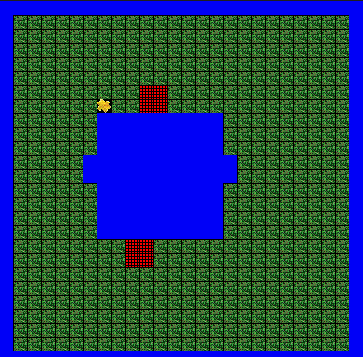
recangular-lake-bump3B.jev ( NOT BEING GRADED, SKIP THIS)
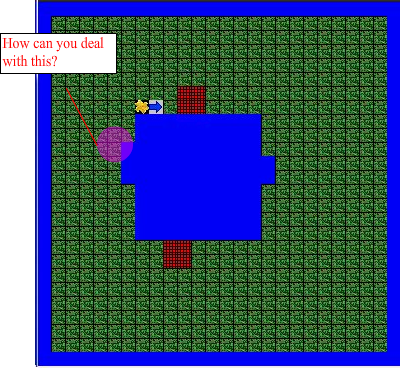
walk-the-lake-jacks-map.jev
Write an infinite loop (1 while loop). You may NOT use isWater()
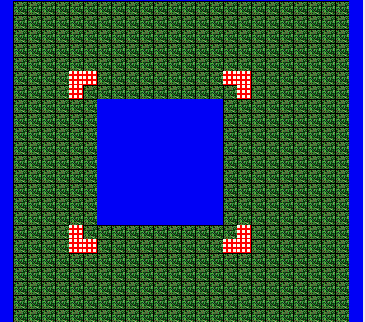