A PlayList project will be based on 3 Classes
The Artist class will store core information about an artist.
The Song class will store basic information about a song.
The PlayList class will manage a list of artists and their ratings.
Tester files:
- Artist and Song tester file SongArtistTester.v0 – run this after you have completed the Song and Artist classes and before PlayList. This way, you’ll know that you have correctly done the the foundation classes before moving onto PlayList
- Some things about grading.
- do not use any external libraries aside from the
ArrayList
- use proper naming conventions for instance variables, for methods and static methods
- make sure that you employ code re-use. (do not copy and paste code, but use methods that you have already written)
Artist
- Constructor(s) :
-
public Artist(String name)
- Instance Variables
-
private String artistName ;
-
private ArrayList<Song> songs ; – an array storing the songs of this artist
- Methods
-
public void addSong(Song song) . Create a song object (see above) and add it to the arraylist. Here’s the first line.
-
public String toString()
-
public boolean equals(Artist other) //returns
true if the names (only) are the same
-
public ArrayList<Song> getSongs()
-
public String getName()
Example of instantiating an Artist object and calling one of its methods:
|
Artist redHotChilliPeppers = new Artist("Red Hot Chili Peppers") ; redHotChilliPeppers.addSong( new Song("Snow") ); ArrayList<Song> chiliSongs =redHotChilliPeppers.getSongs() ; |
Song
- Constructor(s):
-
public Song( Artist _artist, String _name)
- Instance Variables
-
private Artist artist
-
private String name
- Methods
-
public String getName()
-
public Artist getArtist()
-
public boolean equals(Song other) //returns
true if both the song objects have the same artist and name.
-
public String toString()
Example of instantiating a Song object :
|
Artist redHotChilliPeppers = new Artist("Red Hot Chili Peppers") ; Song underBridge = new Song (redHotChilliPeppers , "Under the Bridge") ; |
PlayList
- Static Variable
-
public static final int MAX_NUMBER_SONGS= 6 ;
Instance Variables
-
private String listName ; This is the ‘name’ of your playList
-
private ArrayList<Song> songs ;
-
private ArrayList<Integer> stars ;//how many stars each song has
- Note: songs and stars are parallel ArrayLists
- Constructor
-
public PlayList(String name) : There should be only 1 constructor that takes a parameter representing the name of the playList
- Accessor Methods
-
public double averageRating() // returns the average star rating for the list
-
public ArrayList<Song> getSongs() // returns the songs
-
public double averageRating(Artist artist) // returns the mean star rating associated with artist
-
public Song[] getSongs(Artist artist) // returns an array populated by the songs of parameter artist
-
public ArrayList<Artist> getArtist(String songName) // returns an ArrayList of all Artists associated with the String songName (This could be multiple musicians. Cover songs etc..)
-
private int indexOf(Song someSong) //
-
public String toString() //returns an appropriate String representation of the PlayList . Here is an example of the toString() value for a PlayList I created.
-
public String getName() // @returns the listName of the PlayList
-
public ArrayList<Integer> getStars() //returns the
stars ArrayList
Mutator Methods
-
public void swap(Song song1 , Song song2, ) // switches positions of these two (maintain parallelism!)
-
public boolean add(Song _song , int _stars)
//adds data if number of song is less than MAX_NUMBER_SONGS
-
public void removeSong(Song song) /removes all occurrences of
song . There could be multiple instances of song. Maybe someone really likes a particular song and has it several times in one list. (Hint : Do not loop forwards)
-
public void removeArtist(Artist artist ) //removes all elements associated with
artist
-
public void removeLowStars(int cutOff) //removes all elements associated with a star rating less than or equal to
cutOff
The method’s below are extra credit. If you figure out how to do them, you must also meet with me after school about the methods. To receive the extra credit, be prepared for a mini-quiz on how they work. Be prepared for me to ask about your solution and how changing certain parts of your code would affect your solution. This meeting must happen on the Monday or Wednesday following the deadline.
- **
public PlayList sortByRating() //this returns a rearranged playlist so that the 5 starred elements are the first group in the list, 4 stars second …1 stars, last
- To do this , you should use a sorting algorithm. I suggest, Selection Sort (link). As this both intuitive and on the AP.
- **
public PlayList shuffle()/ /this returns a new PlayList in which all of the songs have been reordered randomly
When you’re done this, begin exploring different sorting algorithms with this online sorting detective :
https://labs.penjee.com/sort-detective/
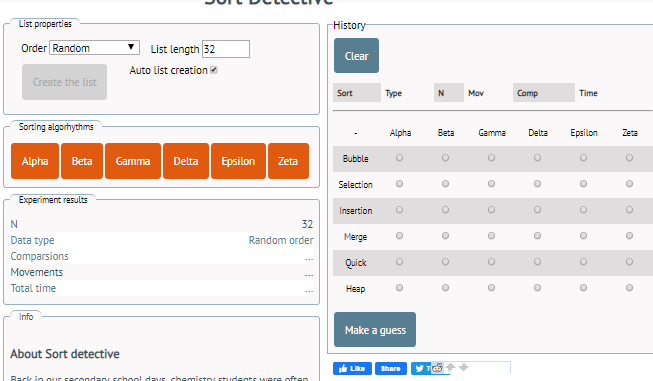