Let’s write a program for something like PowerSchool that helps organize a seating chart for students. There is a tester file at the page’s bottom.
First class :
Student , from https://mrmonline.org/student-class/
Class
SeatingChart
This class mainly manages a 2-d array of student objects. Note: an empty seat in the chart will be designated by a
null value.
|
private Student[][] chart ; // rows and columns for where kids are private String rosterName; //name of this roster private String teacherName; private int period ; //which period is this chart associated public static final int DEFAULT_ROWS = 10; public static final int DEFAULT_COLS = 10; |
Constructor(s)
-
public SeatingChart(int per, String teacher, String _rosterName) Initializes parameters to respective arguments and creates a 2-d array of
null objects with default dimensions
DEFAULT_ROWS , DEFAULT_COLS
-
public SeatingChart(int per, String teacher, String _rosterName, Student[][] table)
Methods:
public String getTeacherName() // returns
teacherName
public String getRosterrName() // returns
rosterName
public int getPeriod() // returns
period
public int countEmptySeats() ; //returns the number of empty seats. As noted earlier, an empty seat is represented by a
null value in the 2-d array of
student s
Student[][] getStudentChart() @returns
chart
public int[] indexOf(Student stu); returns a 2 element array representing the row and column position of
stu in
chart ; [-1 , -1] should be returned if
stu is not in
chart
public boolean setSeat(int row, int col, Student stu) - places
Student Stu at that
row and
column . This can only be done if there is no student in that location. If a student already inhabits that row and column then the method should return
false and not make any changes to
chart .
public void swap(Student s1, Student s2) // this swaps the locations of
s1 and
s2
public Student getStudent(int row, int col) returns the student at the given
row and
col ; or
null if the seat is empty
public void removeStudentAt(int row, int col) sets value of object at
row and
col to
null
public boolean equals(SeatingChart other) // returns
true if all aspects of
other are equivalent to self.
public void colMajorForm() . This prints out the student chart in column major form. Examine the diagram below showing an example seating chart on the left and the printed output on the right.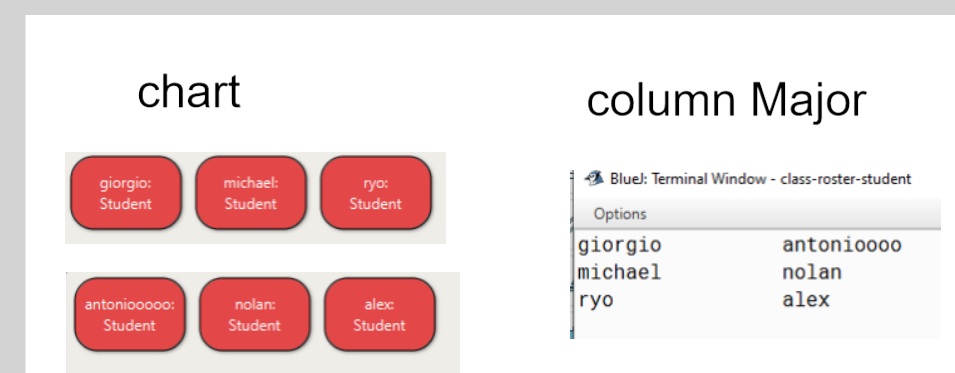
public String toString(); //follow the conventions we have been using. When you print out the chart , let’s show
"E!" t o indicate an empty seat; for seats with students, just display their name.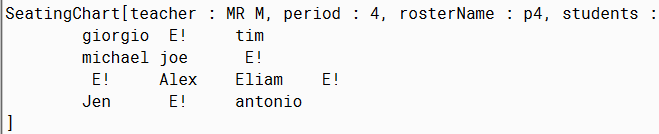
public Student oldest() // returns the student object with the greatest age
Extra credit
public void randomize() // this randomizes the chart. Please be prepared to meet with me to discuss your code. (You cannot use a library to do all the work, if such a library even exists)
SeatingChartTesterv2.java :
import java.util.Arrays;
public class SeatingChartTesterv4
{
public SeatingChartTesterv4()
{
p("v4, null constructor checks");
Student amelia = new Student("Amelia", 17);
Student antonioooo = new Student("antonioooo", 14);
SeatingChart period3 = new SeatingChart(3, "Mr. M", "A Days");
int correct = 0;
int wrong = 0;
boolean didNotInit = false;
if(period3.getTeacherName() == null)
{
p("*non* default constructordid not initialize teacherName");
wrong++;
didNotInit = true;
}
else
correct++;
if(period3.getRosterrName() == null)
{
p("*non* default constructor did not initialize rosterName");
wrong++;
didNotInit = true;
}
else
correct++;
if(period3.getStudentChart() == null)
{
p("*non* default constructor did not initialize chart");
wrong++;
didNotInit = true;
}
else
correct++;
if ( didNotInit == true)
{
throw new Error("You must fix your constructor (non default) before doin anything else \n\t exiting tester");
}
// now let's do the same for default constructor
//SeatingChart(int per, String teacher, String _rosterName
SeatingChart dfault = new SeatingChart( 2, "Mr. Lorensax", "periods 2 ");
if(dfault.getRosterrName() == null)
{
p(" default constructor did not initialize rosterName");
wrong++;
didNotInit = true;
}
else
correct++;
if(dfault.getStudentChart() == null)
{
p(" default constructor did not initialize chart");
wrong++;
didNotInit = true;
}
else
correct++;
if ( didNotInit == true)
{
throw new Error("You must fix your constructor ( default) before doin anything else \n\t exiting tester");
}
//end non default constct test
Student[][] currentChart = dfault.getStudentChart();
if(currentChart[0].length == SeatingChart.DEFAULT_COLS && currentChart.length == SeatingChart.DEFAULT_ROWS)
correct++;
else
{
p("*default constructor's 2-d Array incorrect dimensions");
wrong++;
}
boolean result = period3.setSeat(5,6, amelia );
if(result)
correct++;
else
{
p("setSeat() return value error");
}
Student[][] chartNow = period3.getStudentChart();
if(chartNow[5][6] == amelia)
{
correct++;
if( period3.getStudent(5,6) == amelia)
correct++;
else
{
p("Error, looks like getStudent() ");
}
}
else
{
p("A) erorr, probably from setSeat() or getStudentChart() ");
wrong++;
}
int[] locaxn = period3.indexOf(amelia);
if(locaxn[0]==5 && locaxn[1] == 6)
correct++;
else
{
p("B) setSeat() or indexOf() error");
wrong++;
}
result =period3.setSeat(5,6, antonioooo );
if(result== false)
correct++;
else
{
p("C) setSeat() return value error");
wrong++;
}
String st = period3.toString();
// System.out.println(st) ;
if(st.indexOf("SeatingChart") == 0)
correct++;
else{
p("toString() error. ClassName should be first characters");
wrong++;
}
if(st.contains("Mr. M"))
correct++;
else{
p("toString() error. ");
wrong++;
}
if(st.contains("A Days"))
correct++;
else{
p("toString() error. ");
wrong++;
}
//new seating chart
Student tim = new Student("tim");
Student[][] someKids= {
{ new Student("giorgio"),null, tim , },
{ new Student("michael"), new Student("joe", 12) , null },
{ null, new Student("Alex"), new Student("Eliam", 999), null},
{ new Student("Jen"),null, new Student("antonio", 5), },
};
SeatingChart period4 = new SeatingChart(4, "MR M", "p4", someKids);
//System.out.println( period4 );
//make sure that someKids was actually set to the chart
if(period4.getStudentChart() == null)
{
wrong++;
p("Non default constructor's chart not initialized");
}
else
correct++;
Student[][] p4Chart = period4.getStudentChart();
if( Arrays.equals(p4Chart , someKids))
correct++;
else
{
wrong++;
p("Error . Looks like you did not correctly instantiate 2-d array in constructor ");
}
boolean isCorrect = true;
Student oldest = null ;
try{
oldest = period4.oldest();
}
catch(NullPointerException e){
isCorrect = false;
p("Error @ oldest(), you cannot call .getAge() on a null object");
}
if(isCorrect && oldest != null && oldest.getAge() ==999)
correct++;
else
{
wrong++;
p("Error @ oldest()");
}
locaxn = period4.indexOf(tim);
if(locaxn[0]==0 && locaxn[1] == 2)
correct++;
else
{
p("A incorrect student. Could be constructor");
wrong++;
}
if ( period4.getStudent(0,2) == tim )
correct++;
else
{
p("B incorrect student. Could be constructor, getStudent()");
wrong++;
}
///
int emptySeats = period4.countEmptySeats();
if(emptySeats == 5)
correct++;
else
{
p("countEmptySeats() error");
wrong++;
}
Student vinnie = new Student("Vinnie", 16);
Student yuya = new Student("Yuya", 18);
Student[][] p5Kids = {
{ yuya, vinnie}
};
SeatingChart period5 = new SeatingChart(4, "MR M", "p4", p5Kids);
int[] yuyaLocation_befo = period5.indexOf(yuya);
int[] vinniaLocation_befor = period5.indexOf(vinnie);
period5.swap(vinnie,yuya) ;
int[] yuyaLocation_now = period5.indexOf(yuya);
int[] vinniaLocation_now = period5.indexOf(vinnie);
if( yuyaLocation_now[0] == vinniaLocation_befor[0] && yuyaLocation_now[1] == vinniaLocation_befor[1] )
correct++;
else
{
p("Error Swap");
wrong++;
}
if( vinniaLocation_now[0] == yuyaLocation_befo[0] && vinniaLocation_now[1] == yuyaLocation_befo[1] )
correct++;
else
{
p("Error Swap");
wrong++;
}
////
//
Student rany = new Student("rany", 16);
Student[][] p6Kids = {
{ rany, null,null,null},
{ null, null,null,null}
};
SeatingChart period6 = new SeatingChart(4, "MR M", "p4", p6Kids );
period6.removeStudentAt(0,0);
if( period6.getStudent(0,0) == null )
correct++;
else
{
p("Error @ removeStudentAt() ");
wrong++;
}
if( period5.equals(period6) == false)
correct++;
else
{
p("A Error @ equals() ");
wrong++;
}
if( period5.equals(period5) )
correct++;
else
{
p("B Error @ equals() ");
wrong++;
}
SeatingChart period6Clone = new SeatingChart(4, "MR M", "p4", p6Kids );
if( period6.equals(period6Clone) )
correct++;
else
{
p("C Error @ equals() ");
wrong++;
}
p("----------------------------------");
p("Correct : " + correct);
p("Wrong : " + wrong);
}//end of constructor
void p(String x) { System.out.println(x); }
void p(int x) { System.out.println(x); }
void p(boolean x) { System.out.println(x); }
void p(double x) { System.out.println(x); }
void p2d(int[] x) { System.out.println(x[0] + ", " + x[1]); }
}
SeatingChartTester