Write the body for the methods described below.
Tester File here (This one does not yet test the newest method)
As always, do not import any external libraries. All of the coding can be accomplished by using just loops, arrays, strings and variables. Using anything else will lead to total loss of credit on associated methods.
Once you have completed each method, copy and paste the code in this file into your class. Then run the
test() method and you will get feedback on your code. Note: This does not test all the methods. I have added new methods since writing that tester file. Also, please make sure that:
- all methods are spelled/capitalized exactly as shown on this page
- variables are camelcase and have meaningful names
- for
i loops use i as a variable
-
for-each loops do not use
i as the variable
For each method below, you can assume that the parameters are not
null
Part I
New Method 11.16 – Tester file updated 11.17
boolean isAfterN(String str, String chrs, int index)
Description: This method returns
true if
chrs appears in
str after
index .
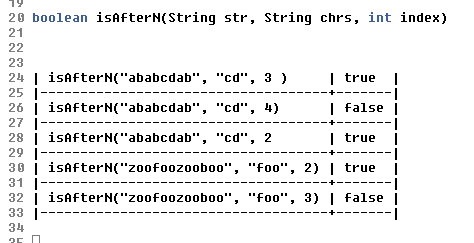
int countF(String str)
Description: This method returns the number of times that the the lower case letter ‘f’ occurs in
str .
Method Call |
return value/output |
countF(“abcdef”) |
1 |
countF(“abcdfef”) |
2 |
countF(“fff”) |
3 |
countF(“xxx”) |
0 |
boolean hasN_Fs(String str, int n )
Description: This method returns true if
str has exactly
n occurrences of the letter ‘f’ in it.
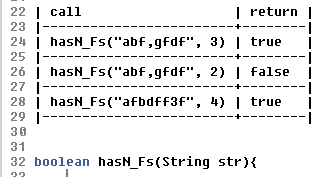
String threeTimes(String str)
Description: This method returns the String str concatenated with itself three times
Method Call |
return value/output |
threeTimes(“foog”) |
“foogfoogfoog” |
threeTimes(“abc”) |
“abcabcabc” |
threeTimes(“zt”) |
“ztztzt” |
threeTimes(“”) |
“” |
String nTimes(String str, int n)
Description: This method returns the
String str concatenated with itself
n times
Method Call |
return value/output |
nTimes(“fg” , 2 ) |
“fgfg” |
nTimes(“abc” , 0 ) |
“” |
nTimes(“zt” , 3 ) |
“ztztzt” |
nTimes(“uiz” , 4 ) |
“uizuizuizuiz” |
int countMiddleChar(String str)
Precondition: str.length() ≥ 3.
Description: This method returns the number of times the middle letter of
str appears in str.
Method Call |
return value/output |
countMiddleChar(“acbcb” ) |
2 |
countMiddleChar(“acbcx” ) |
1 |
countMiddleChar(“bbbbb” ) |
5 |
countMiddleChar(“xytbtzy”) |
1 |
int indexOf(String haystack, String needle)
Description: Write your own indexOf() method. Just to avoid any confusion – you cannot make use of the String’s built in indexOf() method. Our method returns the index of the 1st occurrence of
needle in
haystack . (Full credit if you can get this to work with Strings whose length is greater than 1).
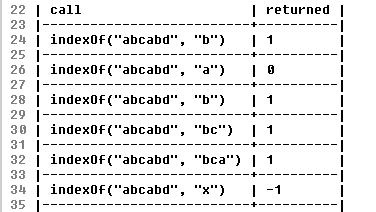
int countChars(String str, String chars)
@precondtion :
chars.length() <= str.lenght()
Description: This method returns the number of times that
chars occurs in String
str .
Method Call |
return value/output |
countChars(“momdadmom” , “dad” ) |
1 |
countChars(“foobofoo” , “foo”) |
2 |
countChars(“foobofoofoo” , “foo”) |
3 |
countChars(“foobofoofoo” , “xy” ) |
0 |
Part II
String[] toArray(String str)
Description: This method returns an array comprised of the individual characters of
str
Method Call |
return value/output |
toArray(“abc” ) |
{ “a”, “b”, “c”} |
toArray(“xyz” ) |
{“x”, “y”, “z”} |
|
|
String[] toArraySansChar(String str, char ch)
Description: This method returns an array of Strings comprised of single letter strings extracted from the input
String str ; however, we will always skip the
char ch :
|
char c = '3'; String s = String.valueOf(c); |

String[] reversedBy2s(String str)
Description: This method returns an array of Strings comprised of pairs of characters from the input, — in reverse order as shown below:
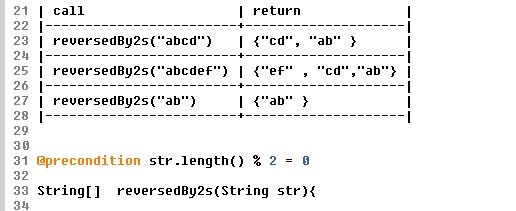
String reverseBy3s(String str)
Description: This is like the prior method, except you are going by 3’s and returning a
String , not an array of Strings.
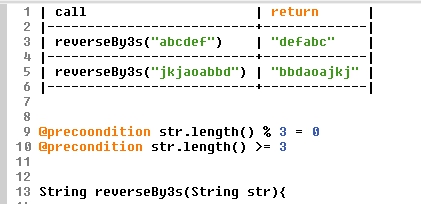
boolean isPalindrome(String str)
Description: This method returns
true if
str is a palindrome
Method Call |
return value/output |
isPalindrome(“abc” ) |
false |
isPalindrome(“aba” ) |
true |
isPalindrome(“abba” ) |
true |