Add the following Methods to a class called ArrayFun2D
public void print(int[][] arr)
Description: This method prints each element in the array. This will be a useful method when you are debugging your code and want to test it out to see if it works.
Method Call | return value |
print({{1,2} , {3,4}, { 5, 6 } } ) | 1 2 3 4 5 6 |
public int sum(int[][] arr)
Description: This method returns the sum of all elements in the array
Method Call | return value |
sum({{1,2} , {3,4}, { 5, 6 } } ) | 21 ( ie 1+2+3+4+5+6) |
public String concat(String[][] strs)
Description: This method returns the concatenation of all the elements in the Array
Method Call | return value |
concat({{“a”,”xc” }, {“t”, “zoo”}, { “jjkj” } } ) | “axctzzoojjkj” |
public double mean( int[][] arr)
Description: This method returns the arithmetic mean of the array. Make sure you double check that you are correctly returning results that include decimal values .
Method Call | return value |
mean({{2,8} , {4 , 7} } ) | 5.25 ie (2+8+4+7)/4 |
public void printRow(int[][] arr, int row)
Description: This method prints all elements in
row
.
This can be (and should be) done with a single loop.
Method Call | return value |
printRow({{1,2} , {3,4}, { 5, 6 } } , 1 ) | 3, 4 |
printRow( { 5 , 6} , {7, 12, 13 , 5 }, { 5, 6 ,0} } , 2 ) | 5 , 6, 0 |
public void printCol(int[][] arr, int col)
Description: This method prints all elements in
col
.
Method Call | return value |
printCol({{1,2} , {3, 4}, { 5, 6 } } , 1 ) | 2 4 6 |
printCol( { 5 , 6} , {7, 12, 13 , 5 }, { 12, 6 ,0} } , 0 ) | 5 7 12 |
printCol( {12, 13 , 5 }, { 6, 2, 13 ,11} , {1,2 } } , 2 ) | 5 13 |
public int maxVal_inRow(int[][] arr, int row)
Description: This method returns the value of the largest number in the given row
Method Call | return value |
maxVal_inRow({{1,2} , {3,4}, { 5, 6 } } , 1 ) | 4 |
maxVal_inRow ({ { 5 , 6} , {7, 12, 13 , 5 }, { 5, 6 ,0} } , 2 ) | 6 |
public int maxVal_inCol(int[][] arr, int col)
Description: This method returns the value of the largest number in the given col
Method Call | return value |
maxVal_inCol({{1,2} , {3,4}, { 5, 6 } } , 0 ) | 5 |
maxVal_inCol({{1,-2} , {3,-4}, { 5, -5 } } , 1 ) | -2 |
public int[][] changeValues(int[][] table, int val)
Description:This method returns the array with all elements set to
val
changeValues(table, 5)
Original Array
1 2 3 4 5 6 Returned Array
5 5 5 5 5 5
public void print_colMajor(int[][] arr)
Description: This method prints each element in the array – using column major order
Method Call | return value |
print({{1,2} , {3,4}, { 5, 6 } } ) | 1 3 5 2 4 6 |
public int[][] removeVals(int[][] table, int val)
Description: This method returns a version of the 2-d array where val has been removed from all elements
removeValues(table, 5)
Original Array
{1 , 5} {3, 4} {5, 6} Returned Array
{1} {3, 4} {6}
int[] twoD_to1D(int[][] arr)
Description: This method takes an input array that is in 2 dimensions and creates a 1 d array . Assume that the array is rectangular (not jagged).
Method Call | return value |
twoD_to1D ( {{1,2} , {3,4}, { 5, 6 } } ) | { 1 , 2 , 3 , 4 , 5 , 6 } |
Matrices
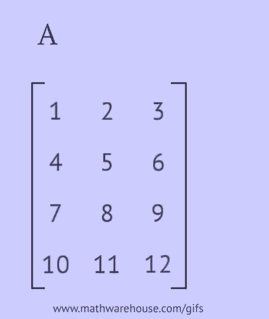
int[][] add (int[][] matr1, int[][] matr2)
Description: This method returns the sum of matr1 and matr2
** int[][] multiply(int[][] matr1, int[][] matr2)
Description: This method returns the product of matr1 and matr2
**public int[][] invertValues(int[][] matr)
Description: This method returns the paramater with its values ‘inverted’
Original Array
1 2 3 4 5 6 Returned Array
6 5 4 3 2 1